Getting to Know JavaScript
Introduction
I’ve been asked to turn you into real hackers, and to teach you how to program in JavaScript. I have two preliminary concepts:
- How does the Internet work
- Why JavaScript?
You may be asking how this is relevant, but perhaps you can trust me, and by the end of the night, you’ll see how.
History of the Internet
Why Do We Learn History?
To understand why things are the way they are.
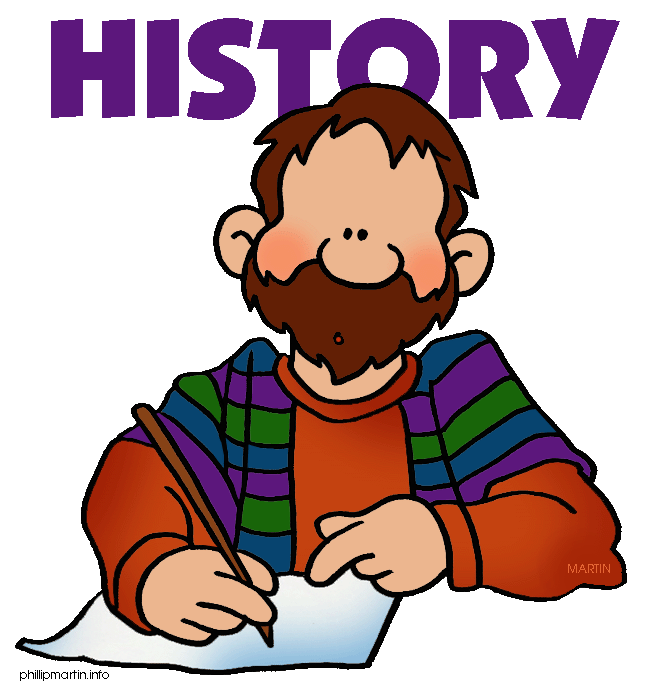
Once upon a time…
- How useful is a single, unconnected computer?
- When I was a kid, to send a program, I used a floppy disk
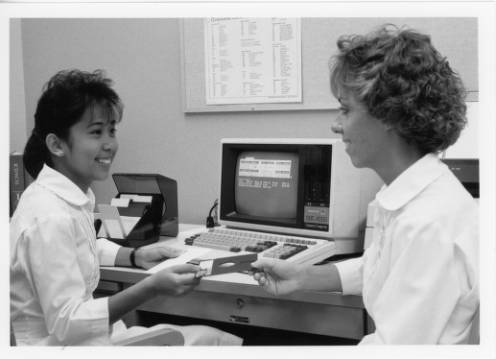
Once Printers were Expensive
Two people (in the same office) could split a printer:
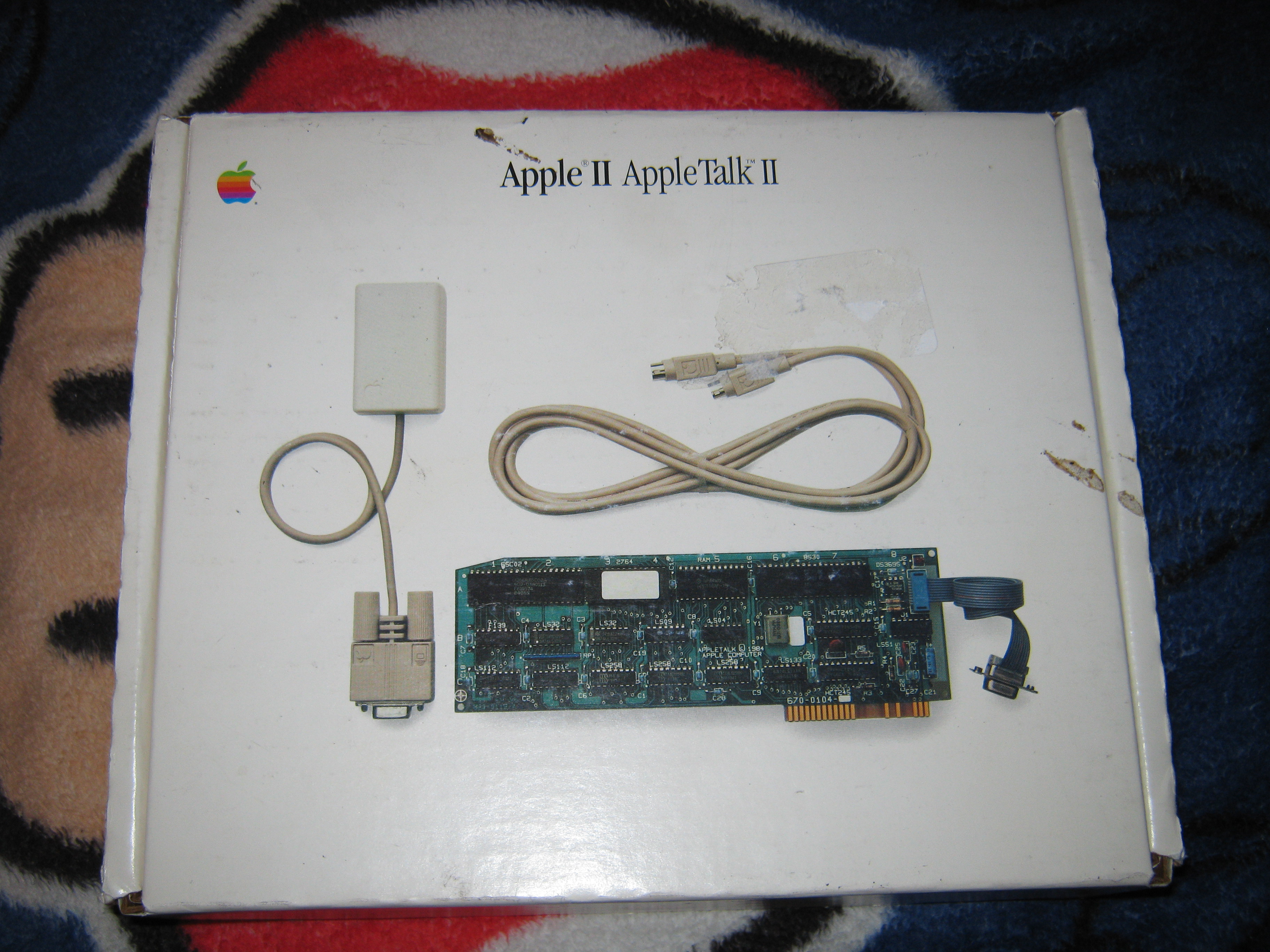
But we Wanted to Talk More!
Computers in 1992
To read her report, while I worked late, Mary had to leave her computer on…
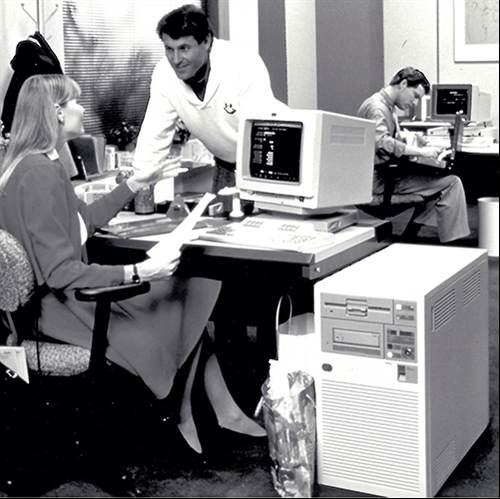
We designated one computer to hold all documents, and we left it on all the time … it would server the documents.
Web Invented in 1993
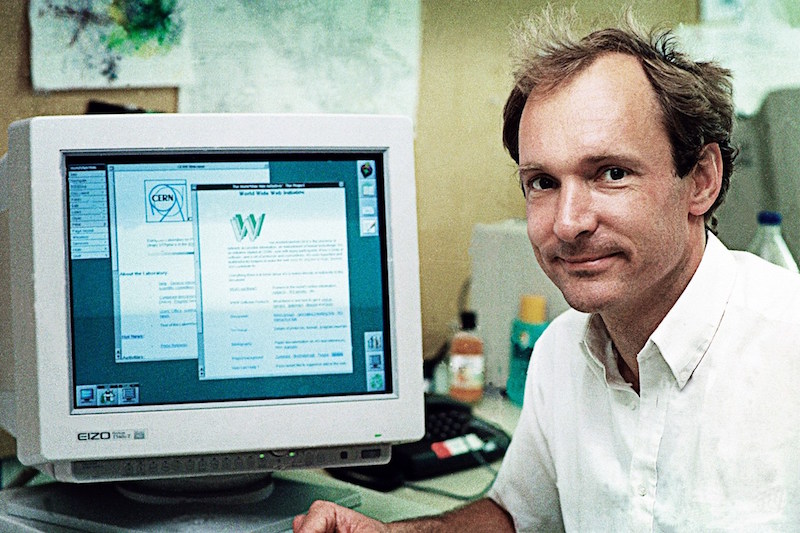
The Greatest Invention
Tim Berners-Lee invented Three Things:
- Easy way to describe a document
- Easy way to fetch a document
- Easy address scheme to find a document on any server
Universal Resource Locator
(URL
)
What is a server?
Two “Types” of Computers
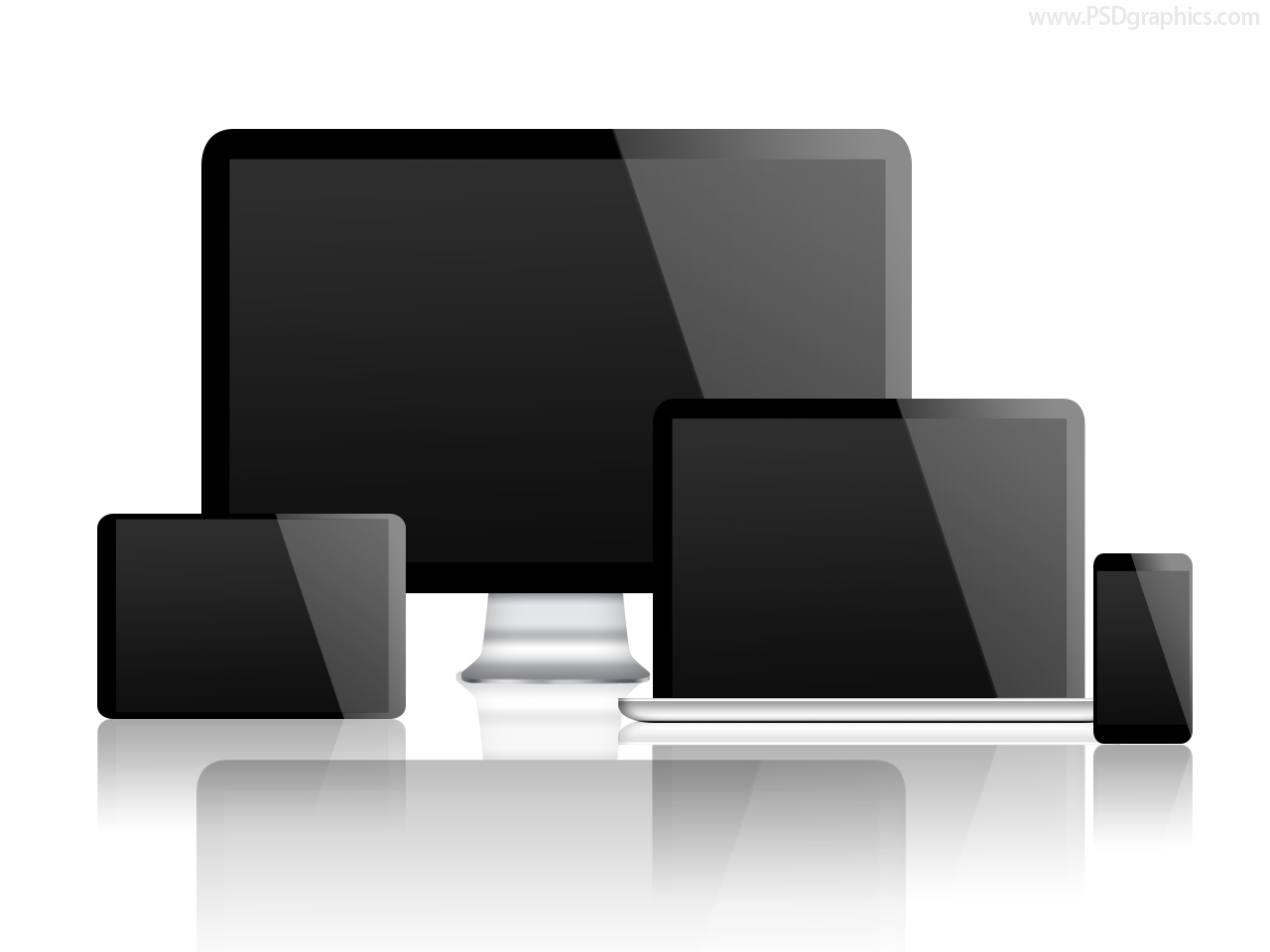
Servers live in Data Centers
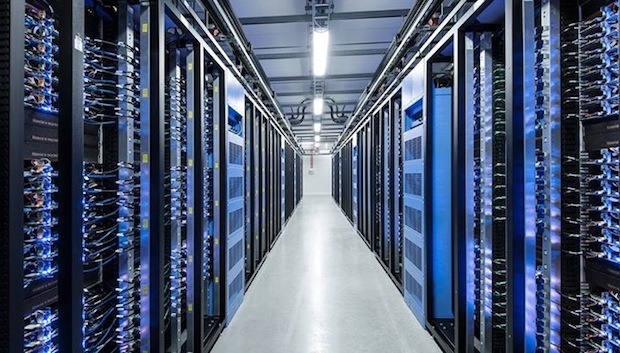
What is a Data Center?
Show a Data Center Tour
Why JavaScript?
Why do you want to learn JavaScript?
Graphical Programming?
- Nice when learning
- Often nice to read
- Slow when you know what you are doing
But how do you distinguish parts of a program?
Programming Syntax
A few different styles. Let’s convert this condition:
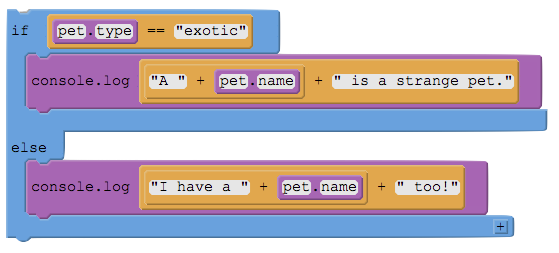
Use Words
We call them key words. This language is called shell:
if [ $pet_type == "exotic" ] then echo "A " $pet_name " is a strange pet." else echo "I have a " $pet_name " too!" fi
Here is the code in Ruby:
if pet.type == "exotic" then puts "A #{pet.name} is a strange pet." else puts "I have a #{pet.name} too!" end
Use Spacing
Blocks contain statements if they are indented underneath. This language is CoffeeScript:
if pet.type == "exotic" console.log "A #{pet.name} is a strange pet." else console.log "I have a #{pet.name} too!"
This is our version in Python:
if pet.type == "exotic": print "A %s is a strange pet." % pet.name else: print "I have a %s too!" % pet.name
Use Fancy Symbols
Conditions and blocks can be surrounded by symbols. This is a language called Lisp:
(if (equal pet-type "exotic") (message "A %s is a strange pet." pet-name) (message "I have a %s too!" pet-name))
The grandfather of many languages was called C.
JavaScript looks a lot like C and Java:
if (pet.type == "exotic") { console.log("A" + pet.name + "is a strange pet."); } else { console.log("I have a " + pet.name + " too!"); }
The Need in 1995
- Web pages were originally static
- We wanted them dynamic
- Two possible approaches:
- The server could dynamically create the document
- The browser could dynamically change it
The Browser Wars
Netscape, a hot, new company in 1994 made the web popular.
Microsoft wanted to own that.
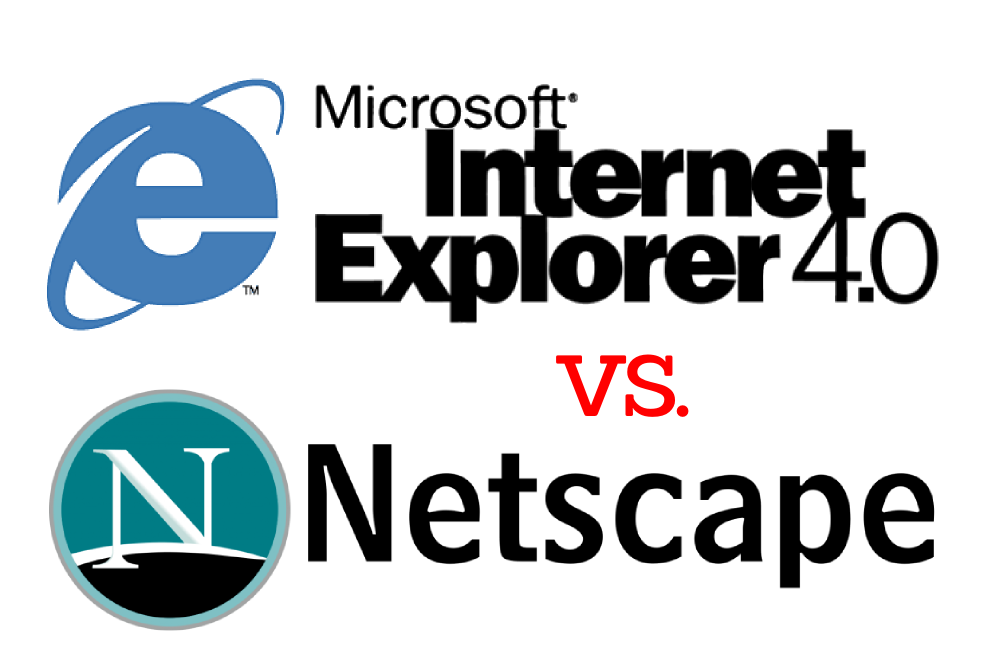
History of JavaScript
Netscape asked Brendan Eich to quickly beat Microsoft by writing programming code in the browser.
He invented JavaScript in 10 days.
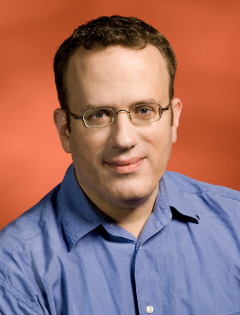
JavaScript is Good…and Bad
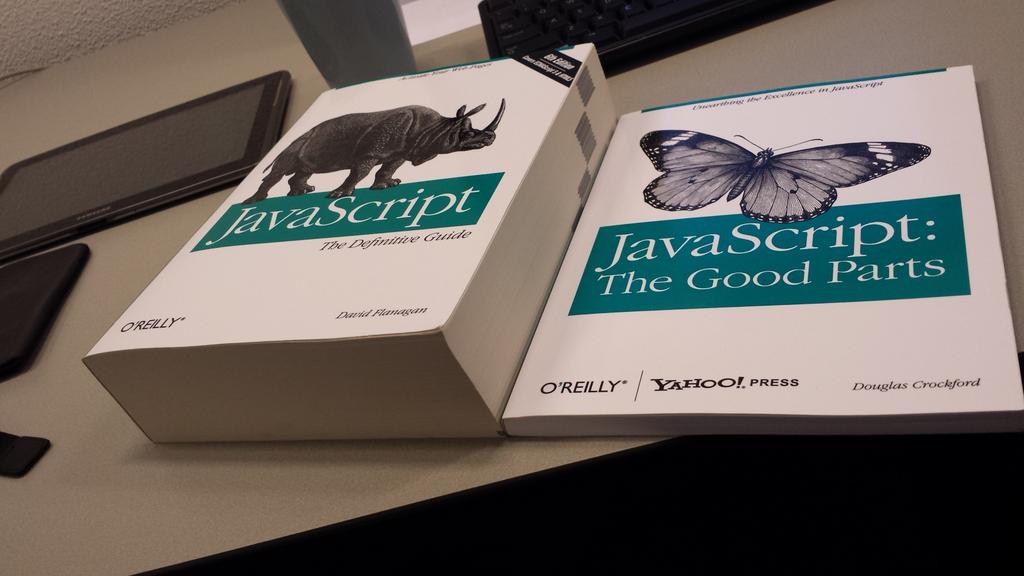
JavaScript Most Used Language
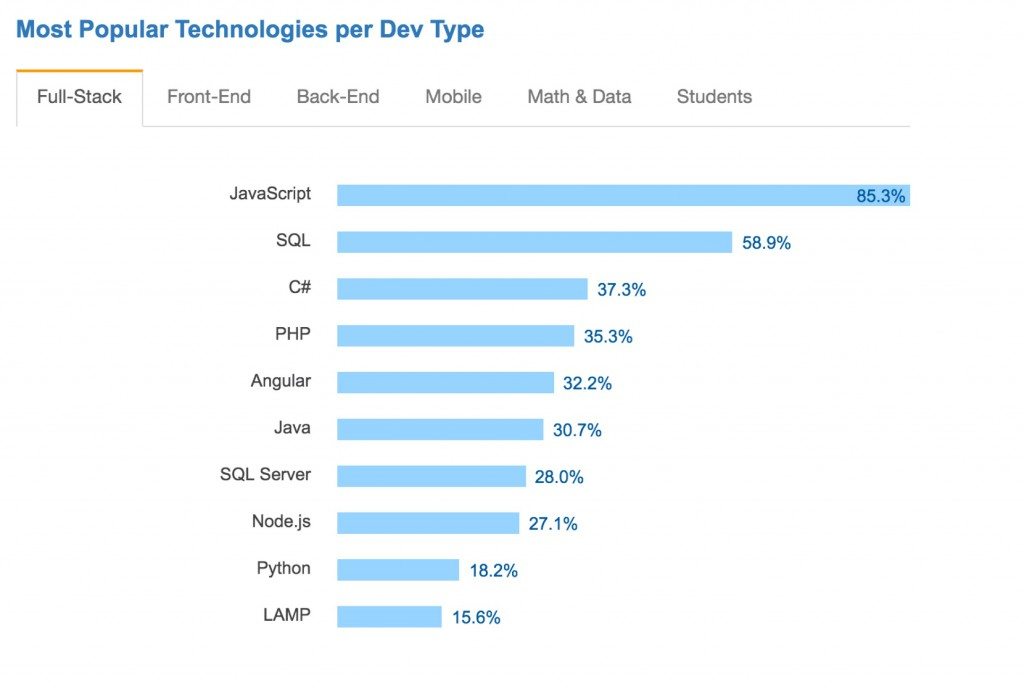
How to Learn JavaScript
Learning Path
- Know the basics of HTML
- Learn bulk of JavaScript
- Know the basics of CSS
- Learn jQuery
Tutorials and Lessons
- Know very little code? Like puzzle learning?
- code.org’s Course 4
- Know some code? Full lesson plan?
- Codecademy’s JavaScript Lessons
- Also has lessons on HTML, CSS and jQuery
- Know some, but you’re impatient?
- W3Schools’ JavaScript Tutorial
- References for HTML and CSS
Need a Project… or, Puzzle
If you can’t figure out a project to focus on, try a puzzle:
Playing Around
- Browser Console and Developer Tools
(type
Command-Option-C
) - GreaseMonkey
- jsfiddle • JS Bin • CodePen
How to Code JavaScript
Write Code
Need an Editor
- Online:
- Local:
- Atom
- Sublime Text
- Eclipse with JSDT
Run Code
Must have an HTML frame:
<html> <head> <script type="text/javascript" src="my-code.js"></script> </head> <body> <!-- Widgets to manipulate --> </body> </html>
Then JavaScript can run:
window.onload="start()"; function start() { // Start my app here... }
Host Code
Remember all that talk about servers?
Need a server to share your work:
- jsfiddle.net
- See me for space on my system